In What is an API we introduced and discussed some of the basics concepts and terms associated with what is defined as an API or Application Programming Interface.
A REST API is an API that conforms to the design principles of the Representational State Transfer (REST) architecture, a software architecture pattern initially created to guide the design and development of the World Wide Web. REST defines a set of constraints for how the architecture of an Internet-scale distributed hypermedia system, such as the Web, should behave. The REST architectural style emphasises the scalability of interactions between components, uniform interfaces, independent deployment of components, and the creation of a layered architecture to facilitate caching of components to reduce user-perceived latency, enforce security, and encapsulate legacy systems.
The REST Architectural style was first defined in 2000 by computer scientist Dr. Roy Fielding in his doctoral dissertation, to provides a relatively high level of flexibility and freedom for developers. This flexibility is just one reason why REST APIs have emerged as a common method for connecting components and applications in a microservices architecture.
In the API Template Pack we discussed some high level technical basics in What is REST, but in this article we will delve a little deeper into the concepts and principles of REST, focusing more on the business perspective of REST.
In What is an API we discussed the concept of an API being an API a mechanism enabling applications or services to access resources within other applications or services. The application or service doing the accessing is called the client, and the application or service containing the resource is called the server.
REST Base API typically follow 6 Design Principles, which are often referred to Architectural Constraints. These constraints are the guiding principles that define the REST architectural style and must be satisfied for an interface to be considered RESTful.
REST API Design Principles
1 . Uniform interface
All API requests for the same resource should look the same, no matter where the request comes from. The REST API should ensure that the same piece of data belongs to only one uniform resource identifier (URI). Resources shouldn’t be too large but should contain every piece of information that the client might need.
2. Client-server decoupling
The client and server applications are completely independent of each other. The only information the client application should know is the URI of the requested resource; it can't interact with the server application in any other ways. Similarly, a server application shouldn't modify the client application other than passing it to the requested data via HTTP.
3. Stateless
REST APIs are stateless, meaning that server does not remember anything about the user who used the API. This means that the server does not store any session data about the client. The client must send all the information that the server needs to fulfill the request. The server will then return a response to the client, but won’t store any session data about the client. This makes REST APIs very fast and scalable.
4. Cacheable
Resources should be cacheable on the client or server side. Server responses also need to contain information about whether caching is allowed for the delivered resource. The goal is to improve performance on the client side, while increasing scalability on the server side.
5. Layered system architecture
In REST APIs, the calls and responses go through different layers. As a rule of thumb, don’t assume that the client and server applications connect directly to each other. There may be a number of different intermediaries in the communication loop. REST APIs need to be designed so that neither the client nor the server can tell whether it communicates with the end application or an intermediary.
6. Code on demand
REST APIs usually send static resources, but in certain cases, responses can also contain executable code. In these cases, the code should only run on-demand.
REST API Resources
The key abstraction of information in REST is a resource. A resource is a conceptual mapping to a set of entities, not the entity that corresponds to the mapping at any particular point in time.
Any information that can be named can be a resource:
- document
- image
- a temporal service (e.g. "today's weather in London")
- a collection of other resources
- a non-virtual object or a digital representation of anything in the real world (e.g. a person)
In other words, any concept that might be the target of a hypertext reference must fit within the definition of a resource, and every resource should have a unique identifier. In the case of HTTP, URIs are used to identify resources.
REST API Methods
There are typically five methods that are commonly used in a REST-based Architecture:
Method | Description |
---|---|
GET | Retrieves a representation of the resource at the specified URI. |
POST | Creates a new resource at the specified URI. |
PUT | Updates the capabilities of the resource at the specified URI. |
PATCH | Updates the capabilities of the resource at the specified URI. |
DELETE | Deletes the resource at the specified URI. |
HEAD | Retrieves the headers of the resource at the specified URI. |
OPTIONS | Retrieves the options available for the resource at the specified URI. |
REST is not about CRUD
Many may intuitively assume that the HTTP method names are used to indicate CRUD based operations, but this is certainly not the case. The idea of an HTTP resource is very abstract and is not really directly related to database design at all.
The HTTP methods are used to indicate the desired action to be performed on the resource. The HTTP methods are not directly related to CRUD operations.
CRUD operations are not a requirement for a REST based API
When to use REST
The REST interface is designed to be efficient for large-grain hypermedia data transfer, optimizing for the common case of the Web, but resulting in an interface that is not optimal for other forms of architectural interaction.
REST API's are great architectural pattern to use when interaction level requires course-grained resources over a HTTP based network. This is common for APIs exposed over the internet that are consumed by web applications, mobile applications or other internet connected devices, where the network latency is high and the bandwidth is low.
REST is defacto standard for exposing APIs over the internet and is the most common architectural pattern used for exposing APIs over the internet. A large majority of organisations have adopted REST as their standard for exposing APIs to develop customer and partner facing Web APIs, and also for exposing internal APIs to support their internal applications.
This is because REST APIs are easy to understand, relatively easy to implement, and easy to consume. REST APIs are also very flexible and can be used to expose a wide variety of data and functionality. REST APIs are also very scalable and performant, and can be used to support a large number of clients. However, unfortunately not all developers or organisations understand the principles of REST which often misuse the term REST to describe any HTTP based API, and worse incorrectly implement their APIs often leading to poor performance and scalability issues, and what is called a REST Anti-Patterns.
What is HATEOAS ?
The term HATEOAS stands for the phrase Hypermedia As The Engine Of Application State, and it is often mentioned synonymously with REST, and is also considered by purists as one of the key principles of REST.
The main idea of HATEOAS is that a client interacts with a RESTful API entirely through dynamically provided hypermedia links, which means that the information provided by the server includes links to the actions that can be taken next. This allows for a more flexible and decoupled system, as clients do not need to have hard-coded knowledge of the API's structure beyond an initial entry point.
In simple terms, with HATEOAS, a client navigates the API through links provided by the server, much like how you navigate a website by clicking on links. This makes the client less dependent on the URL structure of the API.
It was envisioned when HATEOAS was fully implemented within API that the Response object of an API would provide all additional endpoints that were available to interact further with a resource.
The following is an example of how a JSON response might look with HATEOAS in place, the links array provides URIs to various actions related to the resource, such as updating, deleting, or navigating to a related resource.
{
"id": 1,
"name": "Sample Resource",
"links": [
{
"rel": "self",
"href": "/resources/1"
},
{
"rel": "update",
"href": "/resources/1/update"
},
{
"rel": "delete",
"href": "/resources/1/delete"
},
{
"rel": "related-resource",
"href": "/related-resources/5"
}
]
}
Benefits of HATEOAS
- Decoupling: Clients are less dependent on the server's URL structure.
- Discoverability: The API is more self-descriptive and discoverable since clients can explore available actions dynamically through links.
- Evolution: The server can evolve more easily without breaking clients, since the relevant links are provided dynamically.
software design on the scale of decades: every detail is intended to promote software longevity and independent evolution. Many of the constraints are directly opposed to short-term efficiency. Unfortunately, people are fairly good at short-term design, and usually awful at long-term design
Roy Fielding
Problems of HATEOAS
For the most HATEOAS for API's just dont work in practice, and the reality from my experience it is almost never implemented. In fact, I have never seen it implemented in any API's I've integrated with and even API's I've worked on. There are a few reasons for this:
- There are very few good tools to create a REST API using this style
- There are no clients widely used to consume these types of APIs
- Most software teams just never get round to implementing it
- Most teams just rely on documentation to explain how their API's operate.
For the most part, this renders the whole concept of HATEOAS useless. The concepts of loose coupling and evolvability by creating a generic interface are voided when most clients don’t use it.
Over the years I have read many books and blog posts all discussing HATEOAS and listing the benefits of it and how supposedly many organizations implement it in their REST API, but I've never actually seen any evidence of this.
Resource Oriented API's
According to REST API purist perspective, there are actually very few if any pure REST API, primarily because there none that actually implement HATEOAS. For the most part, in my experience, there are only really REST inspired API's, or what are more commonly known as Resource-oriented API's, which are API's that for the most partially implement the typical REST based resource endpoints:
- Create
- Get
- List
- Update
- Delete
In most cases it may be possible that API's may only implement a mixed degree of these types of endpoints for a resource.
For Instance, on some resources you may only have access too GET
& POST
, other resources may only ever have a GET
others will have multiple GET
with a myriad of differing names i.e. GetLatestItem
, GetAllItems
, GetItemByDate
etc
One could argue that these endpoints have been badly designed or poor, but I can tell you from a number of organisations
I've had the opportunity to work with this approach is more common than most care to admit.
resource-oriented APIs rely on the idea of resources which are the key concepts we store and interact with standardizing the things that the API manages
One of best API's I've worked with and appreciate is the Stripe API, for a number of reasons, but mostly because it is a well documented and consistent. Working with API, you'll also notice that there is no HATEOAS implementation, but it uses resource-oriented endpoints to enable interaction.
Top Tip
Resource-oriented APIs are a way of designing APIs to reduce complexity by relying on a standard set of actions called, methods, across a limited set of things, called resources.
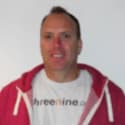
Back-end software engineer
Experienced software developer, specialising in API Development, API Design API Strategy and Web Application Development. Helping companies thrive in the API economy by offering a range of consultancy services, training and mentoring.