In Getting started with nuxt we walked through the basics of creating a web project with nuxt and introduced some of the capabilities of nuxi the Nuxt CLI.
In this guide we are going to delve a little deeper and learn a few additional concepts and processes to use while working with Nuxt, while also implementing Tailwind CSS.
What is Tailwind CSS
Tailwind is define as a utility-first CSS framework. Which sounds great but what exactly does that really geeky phrase mean?
In contrast to other CSS frameworks like Bootstrap or Materialize CSS, Tailwind CSS doesn’t come with predefined components. Instead Tailwind CSS operates on a lower level and provides you with a set of CSS helper classes, using these classes you can rapidly create custom design with ease.
Tailwind CSS is not opinionated and doesn't confine you to component boundaries instead it enables you can create your own unique designs. Tailwind provides a faster UI building process, providing utility classes to build custom designs without the need to write CSS.
Advantages of Tailwind CSS
- No need to define custome CSS classes and Id’s.
- Minimum lines of Code in CSS file.
- customize the designs to create your own components.
- Built to be responsive.
- Makes the changes in the desired manner.
What is even easier is that implementing Tailwind CSS is even easier because we can just use a module to do the work for us.
What is a Nuxt Module
Nuxt provides what is termed as a higher-order module system which basically provides developers the capability to extend the core, without having to edit the core code. Modules are essentially functions that are called sequentially when booting Nuxt.
Nuxt modules can be distributed via npm packages. This makes it possible for them to be reused across mulitple projects and shared with the community, helping to create an ecosystem of high-quality add-ons.
Nuxt itself has been developed using a modular structure and by default ships with a number modules that are already available, we will be touching more on this later in this post.
Adding modules to Nuxt is really easy and there are literally 100's of modules available
Add TallwindCss to your Nuxt Project
The first step to adding TailwindCss to your project is to use nuxi
to add the Nuxt Tailwind
module to your project. The Nuxt TailwindCSS module is an integration that seamlessly incorporates TailwindCSS into
your Nuxt application, enabling rapid and efficient styling of your web projects.
To add the Nuxt TailwindCSS module to your project use the command below.
nuxi module add tailwindcss
This command will not only add the module to your project, but it will also configure it in your nuxt.config.ts
export default defineNuxtConfig({
compatibilityDate: '2024-04-03',
devtools: { enabled: true },
modules: ['@nuxtjs/tailwindcss']
})
What is nuxt.config.ts
The nuxt.config.ts
file is a crucial configuration file in a Nuxt project that defines the global settings and
behaviors for the application. Written in TypeScript, this file enables developers to leverage static typing and type
checking to improve code quality and development experience.
The nuxt.config.ts
file serves multiple purposes:
- configures modules, plugins, middleware, server-side rendering settings, and performance optimizations, among other things.
- centralizes these configurations, it provides a clear and organized way to manage various aspects of a Nuxt application, ensuring consistency and maintainability across the project.
Using TailwindCSS
For the most part your application, will at least at a very basic level be now configured to use TailwindCSS. Let's do a
very simple check to ensure. If you were following on from Getting started with nuxt, we
edited our app.vue
added a very simple greeting, the obligatory "Hello World!". We can now add a little colour to
that by making use of Tailwind, simply edit the file as follows
<template>
<div>
<NuxtRouteAnnouncer/>
<p class="text-orange-500 text-4xl font-bold"> Hello World!</p>
</div>
</template>
View the application in your browser and it will now look something similar to the below.
Nothing too dramatic, but it proves that our application is now configured correctly and we are using Tailwind.
Under the hood, the Tailwind Module does a few things automatically for you, abstracting some of the intricacies of Tailwind configuration for you.
When running yarn dev
, this module will look for these files:
./assets/css/tailwind.css
./tailwind.config.{js,cjs,mjs,ts}
If these files don't exist, the module will automatically generate a basic configuration for them, so you don't have to create these files manually.
Additional tailwind configuration
In most cases, when working with Tailwind you will undoubtedly want to do more advanced styling and configurations. This again can't quite easily be achieved using the CLI etc.
One of the first steps you'll more than likely want to do is add Tailwind Configuration file, namely tailwind.config
and this can be using the npx command below and including the --ts
switch to instruct generating the TypeScript
version of the file
npx tailwindcss init --ts
This will generate the tailwind.config.ts
file in the root of your project directory and will look something similar
too
import type { Config } from 'tailwindcss'
export default {
content: [],
theme: {
extend: {},
},
plugins: [],
} satisfies Config
Conclusion
We have now configured our project to make use of Tailwindcss by adding a custom Nuxt module and configured the
module directly in our nuxt.config.ts
. We can add additional modules, as we progress through this tutorial series
we will continue to do so. However, for now we will leave it here and look forward to the next tutorial in the series.
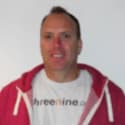
Back-end software engineer
Experienced software developer, specialising in API Development, API Design API Strategy and Web Application Development. Helping companies thrive in the API economy by offering a range of consultancy services, training and mentoring.